Visual C++ - COM Client Application for Internet Explorer - CoCreateInstance
I have come up a COM Client Application that can create an instance for Internet Explorer Control and Naviage to a URL which you type in. I have used CoCreateInstance and I have not used any ATL classes in this sample code.
Source Code
// IEClientDlg.cpp : implementation file
//
#include "stdafx.h"
#include "IEClient.h"
#include "IEClientDlg.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
// CAboutDlg dialog used for App About
class CAboutDlg : public CDialog
{
public:
CAboutDlg();
// Dialog Data
enum { IDD = IDD_ABOUTBOX };
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
// Implementation
protected:
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD)
{
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)
END_MESSAGE_MAP()
// CIEClientDlg dialog
CIEClientDlg::CIEClientDlg(CWnd* pParent /*=NULL*/)
: CDialog(CIEClientDlg::IDD, pParent)
, m_url(_T("www.softwareandfinance.com"))
{
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
void CIEClientDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
DDX_Text(pDX, IDC_EDIT1, m_url);
}
BEGIN_MESSAGE_MAP(CIEClientDlg, CDialog)
ON_WM_SYSCOMMAND()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
//}}AFX_MSG_MAP
ON_BN_CLICKED(IDC_BUTTON1, &CIEClientDlg::OnBnClickedButton1)
END_MESSAGE_MAP()
// CIEClientDlg message handlers
BOOL CIEClientDlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Add "About..." menu item to system menu.
// IDM_ABOUTBOX must be in the system command range.
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
return TRUE; // return TRUE unless you set the focus to a control
}
void CIEClientDlg::OnSysCommand(UINT nID, LPARAM lParam)
{
if ((nID & 0xFFF0) == IDM_ABOUTBOX)
{
CAboutDlg dlgAbout;
dlgAbout.DoModal();
}
else
{
CDialog::OnSysCommand(nID, lParam);
}
}
// If you add a minimize button to your dialog, you will need the code below
// to draw the icon. For MFC applications using the document/view model,
// this is automatically done for you by the framework.
void CIEClientDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, reinterpret_cast<WPARAM>(dc.GetSafeHdc()), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
// The system calls this function to obtain the cursor to display while the user drags
// the minimized window.
HCURSOR CIEClientDlg::OnQueryDragIcon()
{
return static_cast<HCURSOR>(m_hIcon);
}
void CIEClientDlg::OnBnClickedButton1()
{
::CoInitialize(NULL);
IWebBrowser2 *browser = NULL;
if( browser == NULL)
{
HRESULT hRes = CoCreateInstance(CLSID_InternetExplorer, NULL, CLSCTX_LOCAL_SERVER,
IID_IWebBrowser2, (void**)&browser);
if(FAILED(hRes))
{
MessageBox("Failed to create Internet Explorer Control");
return;
}
}
{
VARIANT vEmpty;
VariantInit(&vEmpty);
UpdateData(TRUE);
USES_CONVERSION;
BSTR bstrURL = SysAllocString(A2OLE((const char*) m_url));
HRESULT hr = browser->Navigate(bstrURL, &vEmpty, &vEmpty, &vEmpty, &vEmpty);
if (SUCCEEDED(hr))
{
browser->put_Visible(VARIANT_TRUE);
}
else
{
browser->Quit();
}
SysFreeString(bstrURL);
browser->Release();
browser = NULL;
}
}
Click here to download the VS 2005 Project file and executable
Output
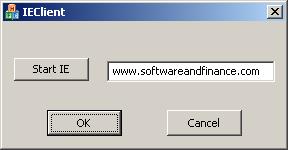
|